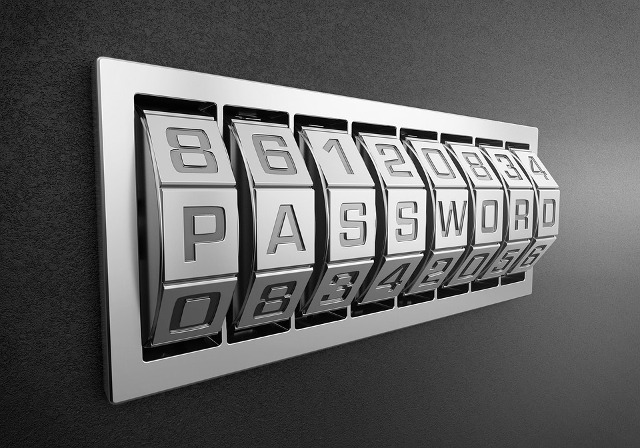
The modern statically typed programming language Golang mainly focuses on concurrency, simplicity, and efficiency. With a design philosophy centered around clean and readable code, Golang provides features for developing robust and scalable applications.
Random strings generate passwords, unique identifiers, and tokens for various purposes. There are mainly two approaches: crypto/rand and math/rand packages. In this blog, we discuss both.
Moreover, in Go applications, generating a random string is a common and unique requirement, from token authentication to generating random identifiers for database records. It ensures strings are more secure in various security and sensitive scenarios. The guide discusses Golang random string with best practices that ensure randomness and security.
Randomness in Go
Go provides facilities to create both pseudo-random and cryptographically secure random numbers. Randomness is a key notion in computer science. Although generated with the math/rand package, pseudo-random integers are inappropriate for security-critical applications. We use the crypto/rand package, which generates cryptographically safe random numbers and ensures confidentiality for these applications.
Utilization of the Math/rand Package
The math/rand package is appropriate when genuine randomization is not required. We can use the deterministic algorithm and seed value to generate a pseudo-random number. Although it is quick and suitable for various tasks, it shouldn’t be used for security-sensitive procedures because it may be predicted if an attacker knows the seed.
Secure Randomness with Crypto/rand
The crypto/rand package is intended for use in security-sensitive applications. It produces cryptographically secure random numbers using platform-specific entropy sources, such as /dev/urandom on Unix-like platforms. In instances like creating secure tokens or encryption keys, when predictability could have detrimental effects, these numbers are appropriate for usage.
Generating Random Strings
You can start by specifying a character set that contains the characters you plan to utilize in Go to produce random strings. For example:
const charset = “abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789”
The next step is to build a function that uses either math/rand or crypto/rand to produce random strings of a given length. Here is a simple math/rand example.
func randomString(length int) string {
rand.Seed(time.Now().UnixNano())
result := make([]byte, length)
for i := range result {
result[i] = charset[rand.Intn(len(charset))]
}
return string(result)
}
And here’s an example using crypto/rand:
func secureRandomString(length int) (string, error) {
bytes := make([]byte, length)
_, err := rand.Read(bytes)
if err != nil {
return “”, err
}
for i := range bytes {
bytes[i] = charset[int(bytes[i])%len(charset)]
}
return string(bytes), nil
}
Always favor the crypto/rand method when creating random strings for security-critical purposes.
Customizing Random String Generation
The character set, intended length, and handling of certain use cases can all be adjusted when creating random strings. For instance, you may design functions that produce random tokens of various lengths or passwords with varied levels of complexity.
Security Best Practices
To ensure the security of your random string generation, follow these best practices:
Use crypto/rand for Security: Always use crypto/rand for security-critical applications to generate random strings.
Strong Entropy Sources: Ensure that your system’s entropy sources are strong and unpredictable.
Secure Storage and Transmission: Safeguard random strings in storage and during transmission to prevent unauthorized access.
Mitigate Security Risks: Be aware of common security risks, such as accidental exposure of tokens, and implement mitigation strategies.
Use Cases From the Real World for Golang random string
Applications in the real world depend significantly on random strings. They are utilized in web applications to create secure session tokens, password reset tokens, and CAPTCHA challenges. Moreover, it is a temporary token in an authentication system for multi-factor or passwordless login.
Take Away
In random string, a task can be achieved in two ways: by math/rand or crypto/rand. Both methods demonstrate Golang’s versatility at different levels of randomness and security requirements. We also cover security risks that are useful in random strings.
In addition, Golang random string generation is essential for many applications, especially those that need security. Moreover, it is crucial to comprehend the difference between cryptographically secure randomness and pseudo-randomness. When security is an issue, always choose crypto/rand and tailor the random string generation to your particular use cases. You can guarantee the integrity and confidentiality of sensitive data in your Go apps by adhering if you hire Golang developers and taking practical applications into account.
Author Bio:
Chandresh Patel is a CEO, Agile coach, and founder of Bacancy Technology. His truly entrepreneurial spirit, skillful expertise, and extensive knowledge in Agile software development services have helped the organization to achieve new heights of success. Chandresh is fronting the organization into global markets in a systematic, innovative, and collaborative way to fulfill custom software development needs and provide optimum quality.